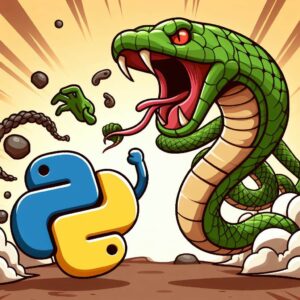
In programming, async (short for asynchronous) refers to a method of executing tasks where actions can occur independently of the main program flow.
Instead of waiting for each task to complete before moving on to the next, async allows the program to continue executing other tasks while waiting for certain operations, such as input/output or network requests, to finish in the background.
Async in python
Python first introduced the capability to use async in Python 3.5 using the async/await keywords, here is a simple example
import asyncio
async def greet():
print("Hello")
await asyncio.sleep(1) # Simulate a time-consuming task
print("World")
async def main():
await asyncio.gather(greet(), greet(), greet()) # Execute multiple async functions concurrently
print("Done")
asyncio.run(main()) # Run event loop
This will produce the following output:
Hello
Hello
Hello
(roughly 1 second later)
World
World
World
Done
Async in JavaScript
This is the equivilent example in JavaScript, one key difference here is you are not in charge of the event loop here like you are with Python.
async function greet() {
console.log("Hello");
await new Promise(resolve => setTimeout(resolve, 1000)); // Simulate a time-consuming task
console.log("World");
}
async function main() {
await Promise.all([greet(), greet(), greet()]); // Execute multiple async functions concurrently
console.log("Done");
}
main();
Promises in javascript
Before the async/await keywords were introduced JavaScript still had promises which more or less achieved the same behaviour in a lower level way with less syntactic sugar, the above await/async method still uses this as its underlying mechanism.
function greet() {
return new Promise((resolve, reject) => {
console.log("Hello");
setTimeout(() => {
console.log("World");
resolve();
}, 1000); // Simulate a time-consuming task
});
}
function main() {
return Promise.all([greet(), greet(), greet()]); // Execute multiple promises concurrently
}
main().then(() => {
console.log("Done");
});
The program flow for this can also feel less linear or “classical” than the more modern async/await approach due to the semantics of how and when the execution of the function is suspended works.
When a Promise is created and the asynchronous operation is started, the code after the Promise creation continues to execute synchronously.
On the other hand, with Async/Await, the await keyword causes the JavaScript engine to pause the execution of the async function until the Promise is resolved or rejected.
For example if we changed the above main() function to look more like the async way you will perhaps get some unexpected results.
function main() {
var p = Promise.all([greet(), greet(), greet()]); // Execute multiple promises concurrently
console.log("this will print before world!")
return p;
}